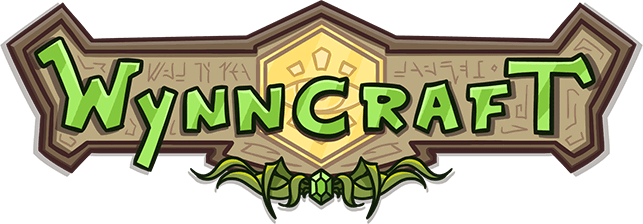
Dismiss Notice

Wynncraft, the Minecraft MMORPG. Play it now on your Minecraft client at (IP): play.wynncraft.com. No mods required! Click here for more info...
Guide XP Bonus Gear (2022) + Script
Discussion in 'Wynncraft' started by LtNifty, Oct 3, 2022.
- Thread Status:
- Not open for further replies.
- Thread Status:
- Not open for further replies.