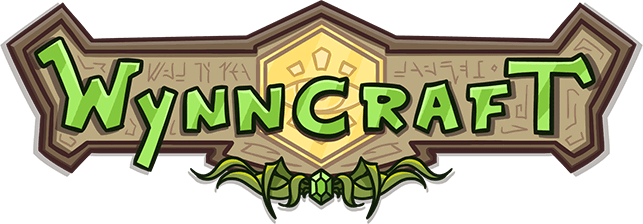
Dismiss Notice

Wynncraft, the Minecraft MMORPG. Play it now on your Minecraft client at (IP): play.wynncraft.com. No mods required! Click here for more info...
SPOILER Wynnscript's Workings [UPDATED!]
Discussion in 'Wynncraft' started by Endistic, Mar 12, 2023.
Tags:
- Thread Status:
- Not open for further replies.
Page 1 of 3
Page 1 of 3
- Thread Status:
- Not open for further replies.